Lamada
函数式接口
概念:有且仅有一个抽象方法的接口
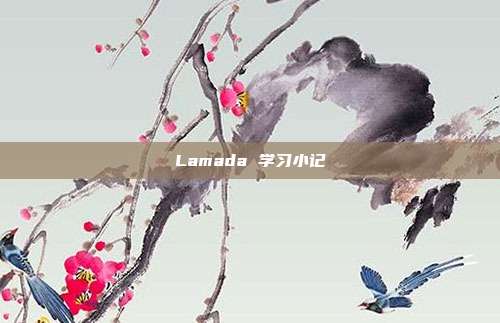
由于只有一个抽象方法 Lamada 才能顺利推导
@FunctionalInterface 放在该接口上强制检查接口是否只有一个抽象方法 否则保存
当然符合有且仅有一个抽象方法也不用加该注解
Lamada 示例
接口中 public abstract 可以省略
@FunctionalInterface public interface MyFunctionalInterface { public abstract void myMethod(String s); }
个人理解为调用接口时重写抽象方法
有返回值示例 - 定义接口
@FunctionalInterface public interface Sumable { int sum(int a, int b); }
定义处理方法
private static void showSum(int x, int y, Sumable sumCalculator) { System.out.println(sumCalculator.sum(x, y)); }
在 Main 方法中调用
showSum(10, 20, (m,n)->m + n);
不写处理方法直接写匿名内部类形式比较容易理解
Sumable sumable = new Sumable() { @Override public int sum(int a, int b) { return a + b; } }; System.out.println(sumable.sum(20, 30));
后一种改成 Lamada 形式
Sumable sumable = (a, b) -> a + b;System.out.println(sumable.sum(20, 30));
函数式编程
Lambda 延迟加载
性能浪费实例
public class Demo01Logger { private static void log(int level, String msg) { if (level == 1) { System.out.println(msg); } } public static void main(String[] args) { String msgA = "Hello"; String msgB = "World"; String msgC = "Java"; log(1, msgA + msgB + msgC); } }
原因
调用方法时候优先拼接了字符串
无论条件是否符合都拼接了
解决
改 Lamada 形式
定义接口
@FunctionalInterface public interface MessageBuilder { String buildMessage(); }
改造方法
public class Demo02LoggerLambda { private static void log(int level, MessageBuilder builder) { if (level == 1) { System.out.println(builder.buildMessage()); } } public static void main(String[] args) { String msgA = "Hello"; String msgB = "World"; String msgC = "Java"; log(1, () ‐ > msgA + msgB + msgC ); } }
类似:SLF4J 会在满足日志级别时进行字符串拼接
LOGGER.debug(" 变量{} 的取值为{}。", "os", "macOS")
Lamada 作参数与返回值
目录
Lamada
- 函数式接口
- Lamada 示例
- 函数式编程
- Lambda 延迟加载
- 性能浪费实例
- 原因
- 解决
- Lamada 作参数与返回值
目录
Lamada
- 函数式接口
- Lamada 示例
- 函数式编程
- Lambda 延迟加载
- 性能浪费实例
- 原因
- 解决
- Lamada 作参数与返回值