最近发现一个奇怪的现象,在国外 Linux 服务器上部署 Java 项目时间与国内 /Windows 上部署时间不一致,我用的是 docker 部署,因此在 Linux 宿主机与 docker 容器中时区都做了更改,但是还是有问题,存在八小时的时差,经多次尝试可以使用以下方式解决:
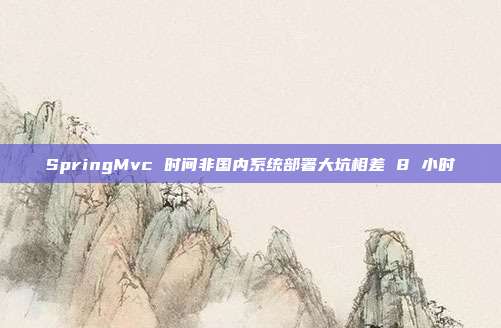
@ResponseBody 注解默认使用 jackson 解析,因此我们可以配置其参数。
对于 SpringBoot,在 spring 下面配置 jackson 相关属性统一处理。
spring: # 设置 jackson 时区 +8 一般系统时间是正确就不需要 (json 时间) jackson: time-zone: GMT+8 # 给返回时间格式化 date-format: yyyy-MM-dd HH:mm:ss
SpringMVC 同理
<mvc:annotation-driven> <!-- 处理请求时返回 json 字符串的中文乱码问题 --> <mvc:message-converters register-defaults="true"> <bean class="org.springframework.http.converter.StringHttpMessageConverter"> <constructor-arg value="UTF-8" /> </bean> <!-- 处理时区问题 --> <bean class="org.springframework.http.converter.json.MappingJackson2HttpMessageConverter"> <property name="objectMapper"> <bean class="com.fasterxml.jackson.databind.ObjectMapper"> <!-- 处理 responseBody 里面日期类型 --> <property name="dateFormat"> <bean class="java.text.SimpleDateFormat"> <constructor-arg type="java.lang.String" value="yyyy-MM-dd HH:mm:ss" /> </bean> </property> <!-- 时区指定 --> <property name="timeZone" value="GMT+8" /> <!-- 为 null 字段时不显示 --> <property name="serializationInclusion"> <value type="com.fasterxml.jackson.annotation.JsonInclude.Include">NON_NULL</value> </property> </bean> </property> </bean> </mvc:message-converters> </mvc:annotation-driven>
当然也可对实体类中单独时间属性进行格式化,例如:
@JsonFormat(pattern="yyyy-MM-dd HH:mm:ss") private Date creatTime;
以上是对返回的 json 进行时区设置,接下来对代码中现在时间操作进行时区获取,最简单的方法:
// 注意大写 HH 是 24 小时制 小写为 12 小时制 static final String TIME_PATTERN = "yyyy-MM-dd HH:mm:ss"; static final String DATE_PATTERN = "yyyy-MM-dd"; /** * 获取东 8 区当前时间 * @return */ public static Date getDate() { ZonedDateTime now = ZonedDateTime.now(ZoneId.of("+08:00")); String formatNow = now.format(DateTimeFormatter.ofPattern(TIME_PATTERN)); Date parse = null; try { parse = new SimpleDateFormat(TIME_PATTERN).parse(formatNow); } catch (ParseException e) { e.printStackTrace(); } return parse; } public static String getDateStr(){ ZonedDateTime now = ZonedDateTime.now(ZoneId.of("+08:00")); return now.format(DateTimeFormatter.ofPattern(DATE_PATTERN)); }
但是最后发现前端居然还是差 8 小时,但查看 post 请求接收的数据是正确的!但是前端框架我也用了 js 格式化日期方法对时间进行格式化!解决这个问题最简单方法使用上述的后端进行格式化时间返回给前端即可,前端不必再进行时间格式化操作。
其实也可以这样
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss", timezone = "GMT+8")
需要引入依赖
<dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.11.2</version> </dependency>
#cmt25