AngularJS 诞生于 2009 年,由 Misko Hevery 等人创建,后为 Google 所收购。是一款优 秀的前端 JS 框架,已经被用于 Google 的多款产品当中。AngularJS 有着诸多特性,最为核心 的是:MVC、模块化、自动化双向数据绑定、依赖注入等等。
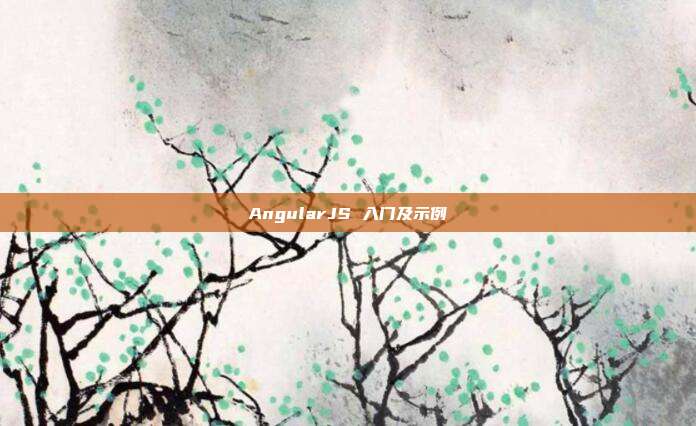
双向绑定(同变量值同步变化):
<html> <head> <title> 入门小 Demo-1</title> <script src="angular.min.js"></script> </head> <!-- ng-init 初始化 --> <body ng-app ng-init="myname=' 蔡徐坤 '"> <!-- {{}}变量表达式调用 --> {{100+100}} <!-- ng-model 变量绑定 双向绑定 即下面同变量名数值都一样 --> 请输入你的姓名:<input ng-model="myname"> <input ng-model="myname"> <br> {{myname}}, 你好 </body> </html>
控制器 controller 与事件:
<html> <head> <title> 控制器 </title> <script src="angular.min.js"></script> <script> var app = angular.module('myApp', []); // 定义了一个名叫 myApp 的模块 []写引入其他模块的名字 // 定义控制器 $scope 作用域 控制层与视图层的数据桥梁 app.controller('myController', function($scope) {$scope.add = function() { // 获取视图层的变量 转换成 int 否则 + 会作为拼接字符串连接符 return parseInt($scope.x) + parseInt($scope.y); } }); </script> </head> <!-- body 绑定模块与控制器 --> <body ng-app="myApp" ng-controller="myController"> x:<input ng-model="x"> y:<input ng-model="y"> 运算结果:{{add()}} </body> </html>
ng-click 事件与 ng-repeat 循环数组与对象数组:
<html> <head> <title> 事件指令 </title> <script src="angular.min.js"></script> <script> var app = angular.module('myApp', []); // 定义了一个叫 myApp 的模块 // 定义控制器 app.controller('myController', function($scope) {$scope.add = function() {$scope.z = parseInt($scope.x) + parseInt($scope.y); } $scope.list = [100, 200, 300]; // 定义数组 /* 循环对象定义 */ $scope.alist = [{ name: ' 张三 ', shuxue: 100 }, { name: ' 李四 ', shuxue: 88 }]; // 定义数组 }); </script> </head> <body ng-app="myApp" ng-controller="myController"> x:<input ng-model="x"> y:<input ng-model="y"> <!-- ng-click 单击事件 --> <button ng-click="add()"> 计算 </button> 结果:{{z}} <!-- 循环数组 ng-repeat = 变量 in 数组 --> <table> <tr ng-repeat="a in list"> <td>{{a}}</td> </tr> </table> <!-- 循环对象 类似 Java 中用点调用对象属性 --> <table> <tr ng-repeat="a in alist"> <td>{{a.name}}</td><td>{{a.shuxue}}</td> </tr> </table> </body> </html>
$http 内置服务:
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title> 内置服务 </title> <meta charset="utf-8" /> <script src="angular.min.js"></script> <script> var app = angular.module('myApp', []); // 定义了一个叫 myApp 的模块 // 内置服务 $http 需要再方法中注入 app.controller('myController', function($scope, $http) {$scope.findAll = function() { // get/post 请求 $http.post('data.json').success(function(response) {$scope.list = response;} ); } // 由于 body 调用了该 controller 因此必定会调用下行方法而执行上述代码 但放 init 中就不必担心别的页面每次调用也会执行 // $scope.findAll();}); </script> </head> <!-- ng-init 除了给变量初始化 也可以调用方法 --> <body ng-app="myApp" ng-controller="myController" ng-init="findAll()"> <table> <tr> <td> 姓名 </td> <td> 数学 </td> <td> 语文 </td> </tr> <tr ng-repeat="entity in list"> <td>{{entity.name}}</td> <td>{{entity.shuxue}}</td> <td>{{entity.yuwen}}</td> </tr> </table> </body> </html>
测试的 json 数据 data.json:
[{"name":" 张三 ","shuxue":100,"yuwen":93}, {"name":" 李四 ","shuxue":88,"yuwen":87}, {"name":" 王五 ","shuxue":77,"yuwen":56}, {"name":" 赵六 ","shuxue":67,"yuwen":86} ]
总结:
var app = angular.module('myApp', []) // 在 js 中定义模块 app.controller('myController', function($scope) {} // 给模块创建名为 myController 的控制器 $scope // 前端与控制层数据的桥梁 ng-app="myApp" // 在 body 标签里绑定模块 将 body 交给 angular 管理 ng-init="findAll() // 初始化变量或者在载入时调用方法 可见第一个 demo ng-controller="myController" // 定义控制器 {{}} // 调用变量或者运算式 <input ng-model="x"> // 为标签绑定变量 ng-click // 单击事件 a in list //in 作为 list 遍历 a.name // 点调用对象属性 $http.post('data.json').success(function(response){return response}) //http 服务发送请求
MVC 模式
首先在 base.js 中创建模块,这里命名为 app
var app=angular.module('tieba',['pagination']); //[] 内为引入的模块 不需要可留空
在服务 js 中写 service:
app.service('tiebaService',function($http){ // 读取列表数据绑定到表单中 this.findAll=function(){ return $http.get('../tieba/findAll.do'); } });
在控制层 js 中写 controller:
// 控制层 app.controller('tiebaController' ,function($scope,$controller ,tiebaService){ // 引用 service 跟内置服务 $controller('baseController',{$scope:$scope});// 继承 baseController // 读取列表数据绑定到表单中 $scope.findAll=function(){ tiebaService.findAll().success( function(response){ $scope.list=response; } ); } });